Redis 实战:Redis 在 Java 中的基本使用
1、使用 jedis 操作 redis
1.1、Jedis 简介
Jedis 是 Java 语言开发的 Redis 客户端工具包,用于 Java 语言与 Redis 数据进行交互。
Jedis 在 github 官网地址:https://github.com/redis/jedis#readme
Jedis 只是对 Redis 命令的封装,掌握 Redis 命令便可轻易上手 Jedis。
Jedis 遵循 RESP 协议规范开发,具有良好的通用性与可读性。
1.2、引入 jedis 的 Maven 依赖
1 2 3 4 5 6
| <!-- https://mvnrepository.com/artifact/redis.clients/jedis --> <dependency> <groupId>redis.clients</groupId> <artifactId>jedis</artifactId> <version>4.4.3</version> </dependency>
|
1.2、获取连接
对于许多应用程序,需要连接到 Redis 时。最好使用连接池。可以像这样实例化一个 Jedis 连接池:
1
| JedisPool pool = new JedisPool("localhost", 6379);
|
对于 JedisPool 实例,可以使用 try-With-resources 块获得连接并运行 Redis 命令(这种方式无须自己手动 close)。
1 2 3 4
| try (Jedis jedis = pool.getResource()) { // 运行单个 SET 命令 jedis.set("clientName", "Jedis"); }
|
1.3、使用实例
编写以下代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| public static void main(String[] args) { JedisPool pool = new JedisPool("127.0.0.1", 6379); try (Jedis jedis = pool.getResource()) { jedis.select(0); jedis.set("Java", "best"); jedis.set("PHP", "good"); System.out.println(jedis.get("Java")); System.out.println(jedis.get("PHP")); } }
|
运行测试用例:

Jedis 实例实现了大多数 Redis 命令,这些命令可以在 https://www.javadoc.io/doc/redis.clients/jedis/latest/redis/clients/jedis/Jedis.htmlApI 中查询命令对应的方法。
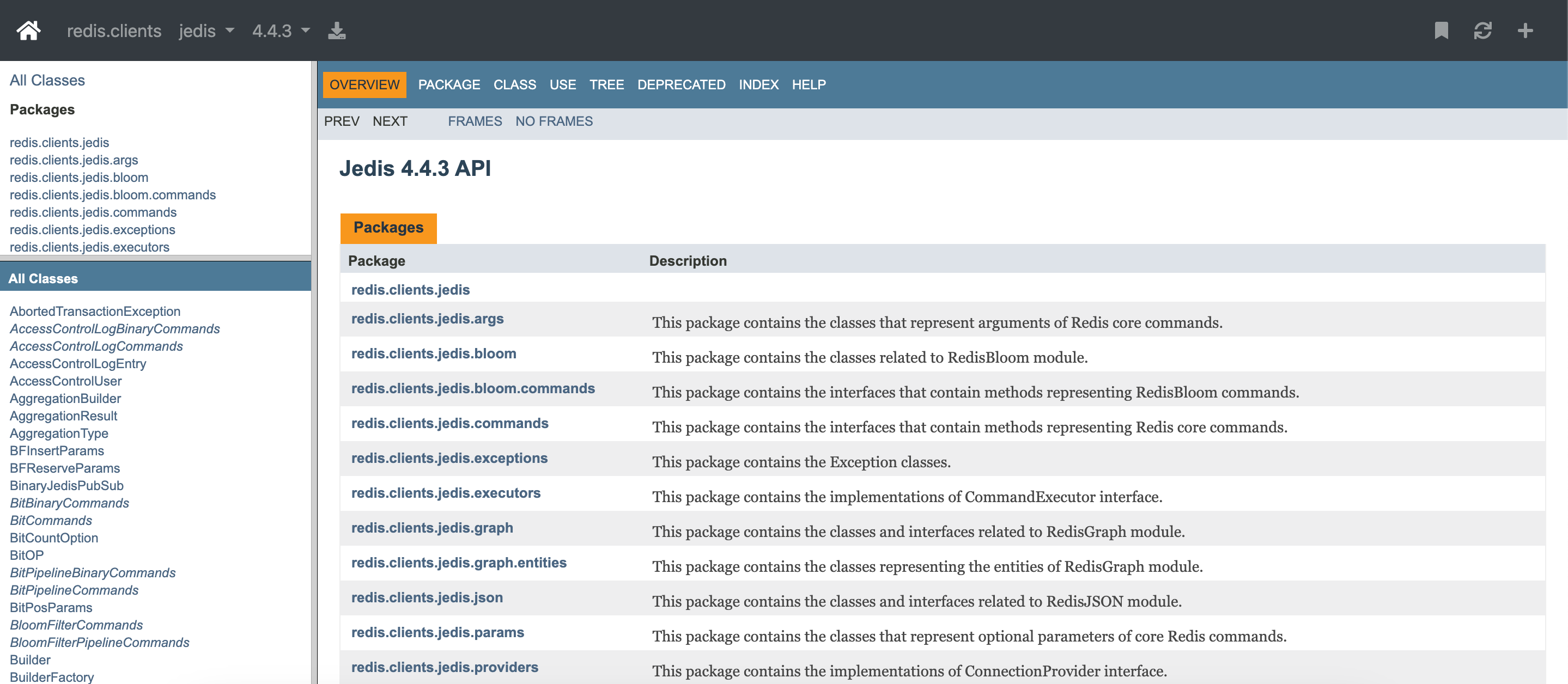
2、对于 JedisPooled 的使用
2.1、使用 JedisPooled
对每个命令使用 try-with-resources 块可能比较麻烦,因此我们可以考虑使用 JedisPooled。
1
| JedisPooled jedis = new JedisPooled("localhost", 6379);
|
详细代码:
1 2 3 4 5 6 7
| public static void main(String[] args) { JedisPooled pool = new JedisPooled("127.0.0.1", 6379, null, null); pool.set("Java", "best"); pool.set("PHP", "good"); System.out.println(pool.get("Java")); System.out.println(pool.get("PHP")); }
|
运行效果:

2.2、关于连接池
使用链接池是官方推荐的使用方式,通过连接池可以更好的使用 Jedis 的,我们可以通过 GenericObjectPoolConfig 对连接池进行相关配置,GenericObjectPoolConfig API 文档:https://commons.apache.org/proper/commons-pool/apidocs/org/apache/commons/pool2/impl/GenericObjectPoolConfig.html
通过 GenericObjectPoolConfig 对象对连接池进行配置,具体代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| public static void main(String[] args) { GenericObjectPoolConfig config = new JedisPoolConfig(); config.setMaxTotal(100); config.setMaxIdle(100); config.setMinIdle(10); config.setTestOnBorrow(false); config.setTestOnReturn(false); config.setTestOnCreate(false); config.setBlockWhenExhausted(true); config.setMaxWaitMillis(1000);
JedisPool pool = new JedisPool(config, "127.0.0.1", 6379);
try (Jedis jedis = pool.getResource()) { jedis.select(0); jedis.set("Java", "best"); jedis.set("PHP", "good");
System.out.println(jedis.get("Java")); System.out.println(jedis.get("PHP")); } }
|
运行效果:

3、SpringBoot 下使用 Redis
3.1、引入 Maven 依赖
首先,需要在 pom.xml 文件中添加 Redis 依赖:
1 2 3 4
| <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency>
|
这个依赖包含了 Spring Data Redis,以及 Jedis 和 Lettuce 这两种 Redis 客户端的实现。
3.2、配置 Redis 连接
在 SpringBoot 项目中,可以通过在 application.properties 或 application.yml 文件中配置 Redis 连接信息。以下是一个示例:
1 2 3 4 5 6 7 8
| spring: data: redis: timeout: 3000 database: 0 password: password port: 6379 host: localhost
|
其中,host 和 port 分别是 Redis 服务器的地址和端口号,password 是 Redis 的密码(如果没有密码,可以不填),timeout 是 Redis 连接超时时间,jedis.pool 是连接池的相关设置。
3.3、创建 RedisTemplate
使用 Spring Data Redis 操作 Redis,通常会使用 RedisTemplate 类。为了方便起见,我们可以创建一个工具类来管理 RedisTemplate 的创建和使用。以下是一个示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123
| import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.data.redis.core.RedisTemplate; import org.springframework.data.redis.core.ValueOperations; import org.springframework.stereotype.Component;
import javax.annotation.Resource; import java.util.Set; import java.util.concurrent.TimeUnit;
@Component public class RedisUtils {
@Resource private RedisTemplate<String,Object> redisTemplate;
private Logger logger = LoggerFactory.getLogger(this.getClass());
public boolean cacheValue(String key, Object value, long time) { try { ValueOperations<String, Object> valueOperations = redisTemplate.opsForValue(); valueOperations.set(key, value); if (time > 0) { redisTemplate.expire(key, time, TimeUnit.SECONDS); } return true; } catch (Throwable e) { logger.error("缓存[" + key + "]失败, value[" + value + "] " + e.getMessage()); } return false; }
public boolean cacheValue(String key, Object value) { return cacheValue(key, value, -1); }
public boolean containsKey(String key) { try { return redisTemplate.hasKey(key); } catch (Throwable e) { logger.error("判断缓存是否存在时失败key[" + key + "]", "err[" + e.getMessage() + "]"); } return false; }
public Object getValue(String key) { try { ValueOperations<String, Object> valueOperations = redisTemplate.opsForValue(); return valueOperations.get(key); } catch (Throwable e) { logger.error("获取缓存时失败key[" + key + "]", "err[" + e.getMessage() + "]"); } return null; }
public boolean removeValue(String key) { try { redisTemplate.delete(key); return true; } catch (Throwable e) { logger.error("移除缓存时失败key[" + key + "]", "err[" + e.getMessage() + "]"); } return false; }
public boolean removeKeys(String pattern) { try { Set<String> keySet = redisTemplate.keys(pattern + "*"); redisTemplate.delete(keySet); return true; } catch (Throwable e) { logger.error("移除key[" + pattern + "]前缀的缓存时失败", "err[" + e.getMessage() + "]"); } return false; }
}
|
在这个示例中,我们使用 @Resource
注解自动注入了一个 RedisTemplate<String, Object>
对象。然后,我们提供了三个方法来对 Redis 进行操作:cacheValue 方法用于缓存数据,getValue 方法用于获取缓存数据,removeValue 方法用于删除缓存数据。这些方法都是通过 redisTemplate 对象来实现的。
需要注意的是,在使用 RedisTemplate 时,需要指定键值对的类型。在这个示例中,我们指定了键的类型为 String,值的类型为 Object。
3.4、使用 RedisTemplate
在上面的示例中,我们已经创建了一个 RedisTemplate 对象,并提供了一些方法来对 Redis 进行操作。现在,我们可以在 SpringBoot 项目中的任何地方使用这个工具类来进行缓存操作。以下是一个示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RestController;
@RestController public class UserController {
@Autowired private RedisUtils redisUtils;
@Autowired private UserService userService;
@GetMapping("/users/{id}") public User getUserById(@PathVariable Long id) {
String key = "user_" + id; User user = (User) redisUtils.getValue(key); if (user == null) { user = userService.getUserById(id); redisUtils.cacheValue(key, user); } return user; } }
|
在这个示例中,我们创建了一个 UserController 类,用于处理 HTTP 请求。在 getUserById 方法中,我们首先构造了一个缓存的 key,然后使用 redisUtils.getValue
方法从 Redis 中获取缓存数据。如果缓存中没有数据,我们调用 userService.getUserById
方法从数据库中获取数据,并使用 redisUtils.cacheValue
方法将数据存入 Redis 缓存中。最后,返回获取到的数据。
通过这个示例,我们可以看到,在 S pringBoot 项目中使用 Redis 作为缓存的流程。我们首先需要添加 Redis 依赖,然后在配置文件中配置 Redis 连接信息。接着,我们创建了一个 RedisUtil s 工具类来管理 RedisTemplate 的创建和使用。最后,我们在控制器中使用 RedisUtils 来对 Redis 进行缓存操作。